Web Scraping Comprehensive Guide: Techniques and Best Practices
Long-Form Informational Article and Actionable Tips To Get Introduced to Web Scraping and start making money or great products. Or both.
- Author: Anna Farekh
- Posted on: November 18th
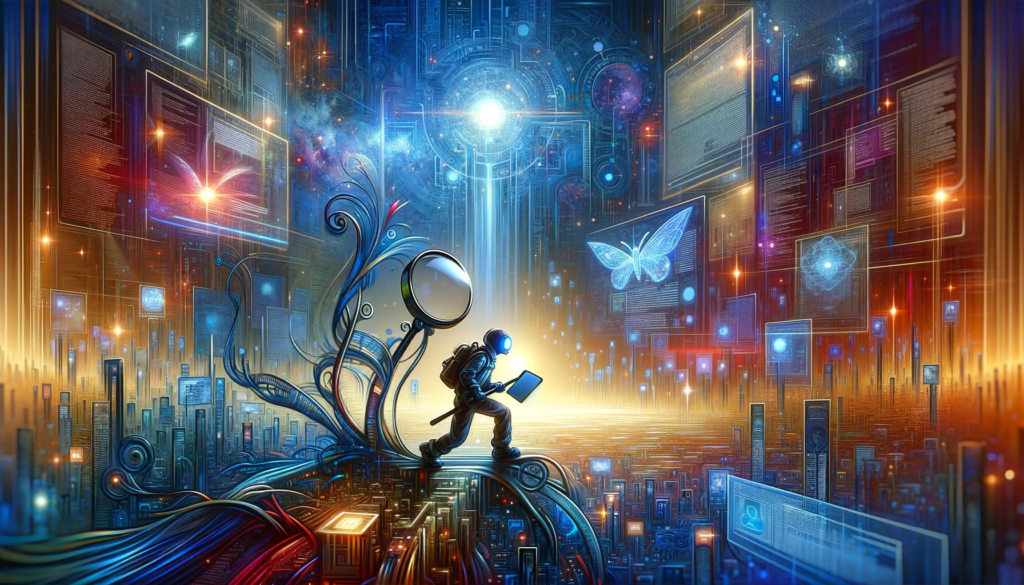
Introduction to Web Scraping
Why is Web Scraping Important?
Web Scraping Requirements
Code Example: Basic Python Scraper
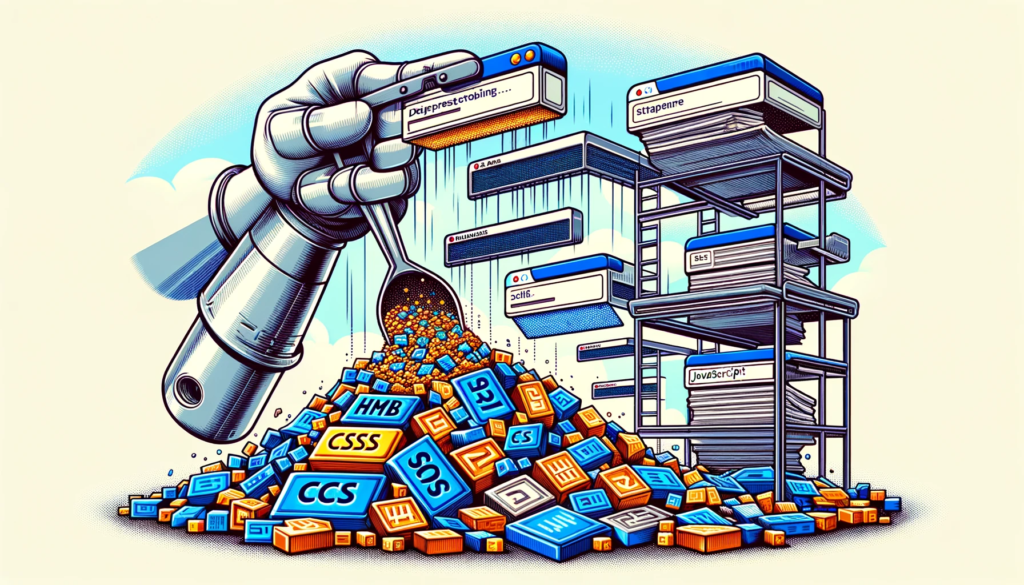
import requests
from bs4 import BeautifulSoup
url = 'http://example-news-site.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for headline in soup.find_all('h2', class_='news-headline'):
print(headline.text.strip())
The Mechanics of Web Scraping
Challenges in Web Scraping
Web scraping isn’t without its challenges. Websites can be complex, with data spread across multiple pages and behind login forms or other interactive elements. Additionally, websites often change their layout or coding practices, which can break your scraping setup. It’s a game of cat and mouse, where webmasters change their site to keep it fresh and functional, and scrapers adapt to these changes to continue extracting data.
Web Scraping Tools (No Code)
An Overview of Web Scraping Tools
Choosing the right tools is essential for effective web scraping. The landscape is rich with specialized software designed to simplify the process. Let’s look at some of the most popular ones:
- Octoparse is a user-friendly, visual scraping tool that’s great for non-coders. It offers a point-and-click interface to select the data you want to scrape, making the process as easy as ordering from a menu.
- Instant Data Scraper is a browser extension that works well for quick and simple scraping tasks. It’s like using a calculator; you get the results instantly without much setup.
- Webscraper.io is another browser extension that allows you to create sitemaps and navigate through the website as a user would, which is ideal for scraping data across multiple pages.
Building or Buying?
The Role of APIs in Web Scraping
Staying Ahead of the Curve
The world of web scraping tools is always evolving, with new software emerging to solve the latest challenges faced by scrapers. Keeping abreast of these developments is crucial to maintain an edge in data extraction.
By understanding the variety of tools available and the considerations for selecting the right one, you can equip yourself with the best means to conduct your web scraping projects. The next section will guide you through the specifics of web scraping using one of the most powerful tools in the field: Python.
Web Scraping with Python
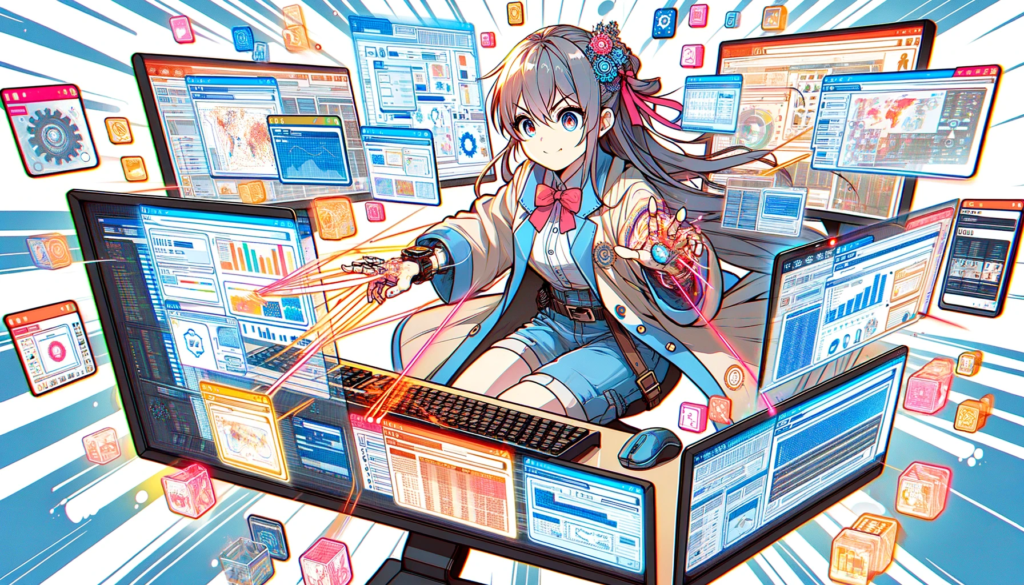
The Python Advantage in Web Scraping
Why Python for Web Scraping?
Python is often recommended for web scraping for several reasons:
- Rich Ecosystem: Python’s ecosystem is replete with libraries designed for web scraping, like Requests, BeautifulSoup, and Scrapy.
- Ease of Learning: Python’s syntax is clear and intuitive, making it accessible to newcomers.
- Community Support: A vast community of Python developers means that help is always available, along with a wealth of tutorials and guides.
A Python Scraping Tutorial
Web Scraping Guide Python
A comprehensive guide to web scraping with Python would cover more advanced topics, such as:
- Handling JavaScript: Many modern websites use JavaScript to load content dynamically. Python scraping tools like Selenium can interact with web pages just like a human user, allowing you to scrape these dynamic pages.
- Dealing with Pagination: Extracting data from multiple pages, or pagination, is a common challenge in web scraping. Python scripts can be written to loop through page numbers and collect data from an entire dataset.
- Managing Sessions and Cookies: Some websites require you to maintain a session or store cookies to access certain data. Python’s Requests library can handle sessions, and you can use it to mimic a logged-in user.
Advanced Python Scraping Techniques
Once you’ve got the basics down, you can explore more sophisticated techniques, such as:
- Asynchronous Scraping: Using Python’s asyncio and aiohttp libraries, you can make asynchronous requests to speed up the scraping process.
- Scraping via APIs: When available, APIs are the preferred method for data extraction. Python provides several libraries, like requests or urllib, to interact with APIs directly.
- Data Cleaning and Storage: After scraping, you may need to clean or process your data before it’s useful. Python excels here with libraries like Pandas, which allow for extensive data manipulation and storage.
Best Practices with Python Scraping
- Respect Robots.txt: This file on websites outlines the scraping rules and which parts of the site should not be scraped.
- User-Agent String: Changing the user-agent string in your request header helps mimic a real browser and can prevent your scraper from being blocked.
- Error Handling: Implement robust error handling to manage issues like network problems or unexpected website changes without crashing your scraper.
By mastering Python for web scraping, you’ll be able to tackle nearly any data extraction project with confidence. The next sections will build on this knowledge, introducing you to real-world applications and the legal framework you need to navigate to scrape responsibly.
Effective Web Scraping Techniques
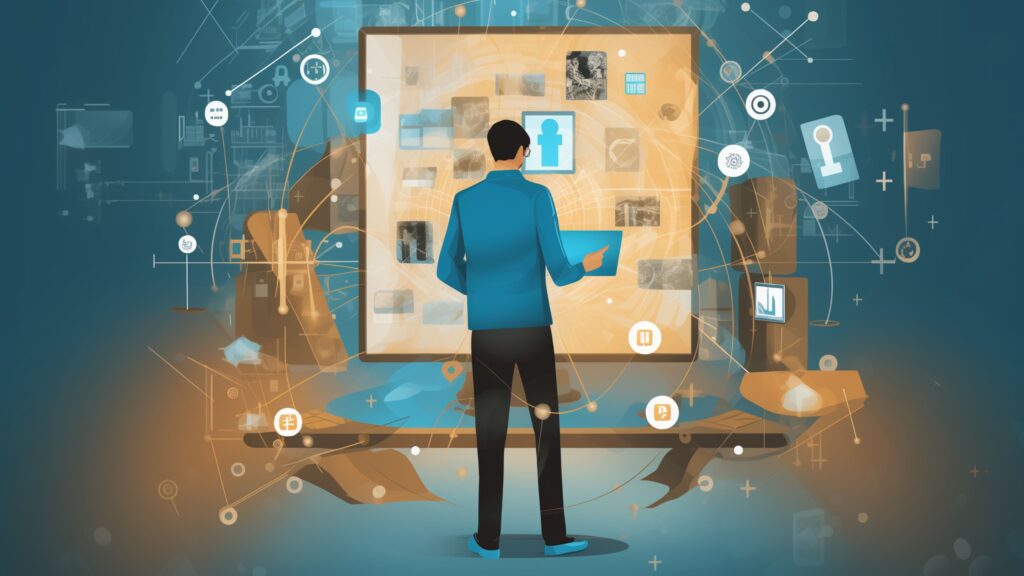
Best Practices for Scraping Data from a Website
To scrape data effectively, you need to adopt a set of best practices that ensure efficiency and minimize the risk of being blocked by the source website. Here are some of the best practices to follow:
- Be Considerate: Make requests at a reasonable rate. Bombarding a site with too many requests in a short time span can overload the server, which may lead to your IP being blocked.
- Stay Under the Radar: Implement delays between your requests and rotate user agents and IP addresses if necessary. This helps mimic human behavior and avoids triggering anti-scraping mechanisms.
- Know Your Source: Understand the structure of the website you’re scraping. This includes knowing when the site’s content is updated and how it’s structured, which can save you from scraping outdated or irrelevant data.
Advanced Web Scraping Resources
As you become more comfortable with basic scraping, you may need advanced resources to tackle more complex tasks. These resources include:
- Web Scraping Frameworks: Frameworks like Scrapy are designed for large-scale web scraping projects. They provide built-in features for handling requests, processing data, and managing concurrency.
- Cloud-Based Scraping Services: Services like Scrapinghub offer cloud-based solutions that can run your scraping jobs without the need for local hardware and software setup.
Effective Web Scraping Techniques
To refine your web scraping skills, consider the following techniques:
- Data Extraction Logic: Develop a strong logic for data extraction, which can handle changes in the website’s layout or content. This often involves writing regular expressions or XPath queries that are robust and flexible.
- Headless Browsers: Use headless browsers for scraping dynamic content rendered by JavaScript. Tools like Puppeteer or Selenium can automate browsers and mimic human interaction with web pages.
- Captcha Solving Services: For sites that use CAPTCHAs to deter bots, consider using CAPTCHA solving services or build mechanisms to manually solve them if the volume is low.
Web Scraping Examples and Use Cases
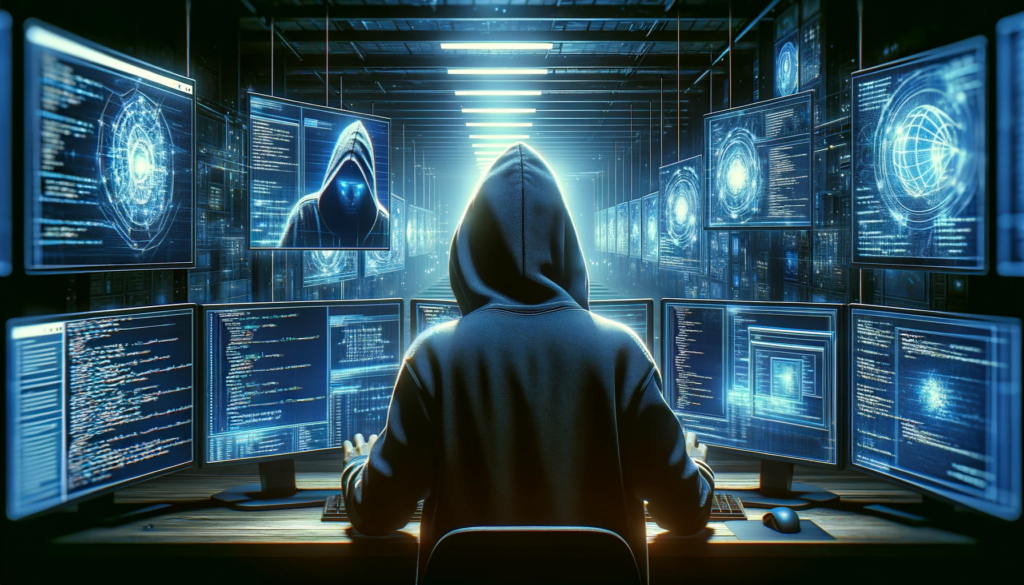
The applications of web scraping are as diverse as the internet itself. Here are some concrete examples where web scraping is used to drive value:
- E-commerce Price Monitoring: Businesses use web scraping to monitor competitor pricing and inventory. By scraping e-commerce sites, companies can adjust their pricing strategies in real time to stay competitive.
- Lead Generation: Marketing agencies scrape websites for contact information, which can be used to build lists of potential leads for sales and outreach campaigns.
- Social Media Analysis: Scraping social media platforms can yield insights into public sentiment about products, services, or brands, informing marketing strategies and product development.
Web Scraping Examples
To illustrate the power and versatility of web scraping, consider these scenarios:
- Travel Fare Aggregation: Web scraping is used to gather flight and hotel prices from various travel portals to provide customers with the best deals.
- Real Estate Listings: By scraping real estate websites, investors can access a large volume of property listings, which can be analyzed to identify investment opportunities.
- News Aggregation: Media and news organizations scrape news from various online sources to aggregate content or conduct analysis on media trends.
Use Cases That Benefit from Web Scraping
Web scraping isn’t just for large corporations. Here are some use cases that show its broad applicability:
- Academic Research: Researchers scrape academic publications, journals, and databases to collect data for literature reviews or data analysis.
- Job Boards: Recruitment agencies scrape job boards to find new postings and aggregate them on their platforms, providing job seekers with comprehensive listings.
- Stock Market Analysis: Financial analysts scrape stock market data to track movements in real-time, allowing for timely investment decisions based on current trends.
How Web Scraping Fuels Business Intelligence
Challenges in Real-World Scraping
Despite its utility, web scraping comes with challenges that must be navigated carefully:
- Data Quality: Ensuring the scraped data is accurate and relevant is crucial. This may involve validating and cleaning data post-extraction.
- Legal and Ethical Boundaries: The legality of scraping public data can be a gray area, and ethical considerations should guide the scraping practice to ensure respect for privacy and intellectual property rights.
- Technical Barriers: Websites with complex navigation structures, anti-bot measures, and large amounts of data present technical barriers that require sophisticated scraping solutions.
Real-World Case Studies: E-commerce Price Tracking
For instance, if a competitor lowers the price of a popular item, ShopFast can respond promptly to match or beat the price. This strategy not only helps ShopFast remain competitive but also provides insights into market trends, which can inform broader business strategies.
Such dynamic pricing models, powered by web scraping, are becoming a staple in the e-commerce industry for maintaining market relevance and competitive edge. By exploring these examples and use cases, we can appreciate the depth and breadth of web scraping’s impact. It’s a practice that, when done correctly, can unlock a wealth of knowledge and opportunities across a multitude of sectors. The following sections will delve into the legal considerations and the cost-benefit analysis of web scraping, providing a comprehensive view of its role in the digital landscape
Legal and Ethical Considerations
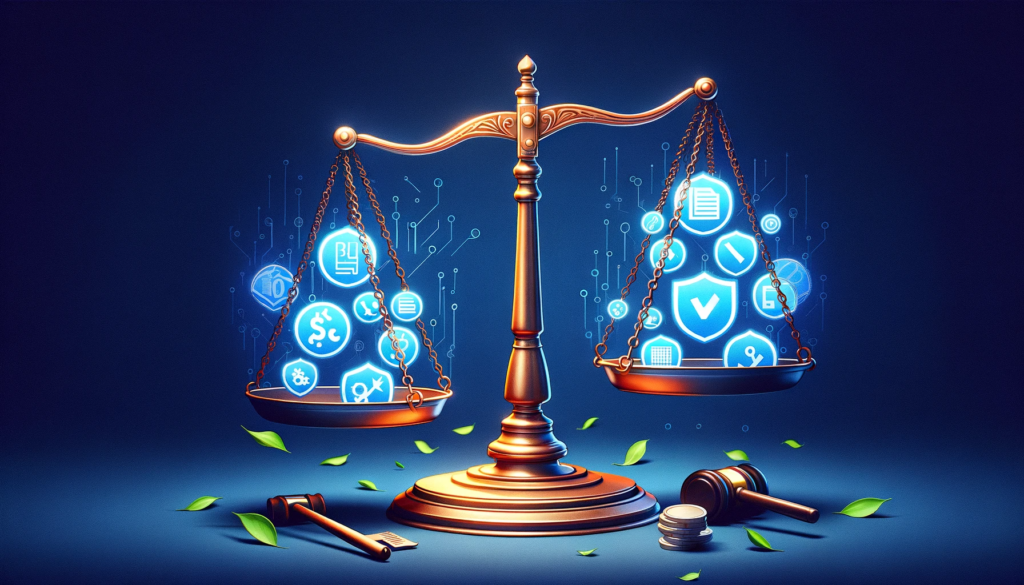
Navigating Web Scraping Rules
Understanding the Legal Framework
The legality of web scraping depends largely on the source of the data, the method of extraction, and the use of the scraped data. Here are some key legal considerations:
- Copyright Law: In many jurisdictions, the content published on the web is copyrighted, and unauthorized reproduction can lead to legal issues.
- Terms of Service (ToS): Violating a website’s ToS can result in banned access or legal action. Many websites explicitly prohibit scraping in their ToS.
- Computer Fraud and Abuse Act (CFAA): In the United States, the CFAA can make unauthorized access to computer systems (which can include scraping) a criminal offense.
Ethical Web Scraping Practices
Ethical scraping is about more than just following the law; it’s about respecting the ecosystem of the internet. Here are some guidelines for ethical scraping:
- Transparency: Be clear about who you are and why you are scraping data. If you’re using it for research, say so.
- Minimize Impact: Design your scrapers to have a minimal impact on the website’s operation. This means not overloading servers or scraping at peak times.
- Data Privacy: Be mindful of personal data. Scraping personal information without consent can breach privacy laws like the GDPR in the EU.
Best Practices for Compliance
To ensure you’re scraping within legal bounds, consider the following best practices:
- Seek Permission: When in doubt, ask for permission to scrape. It’s the most straightforward way to avoid legal trouble.
- Adhere to Robots.txt: This file is a site’s way of communicating which parts of the site you can and cannot scrape. Respecting it is a sign of good faith.
- Avoid Bypassing Protections: If a site has put measures in place to prevent scraping (like CAPTCHA, login requirements, etc.), circumventing these protections can be seen as unauthorized access.
The Consequences of Non-Compliance
Ignoring legal and ethical guidelines can result in serious consequences, from being sued for damages to criminal charges. It’s not just bad for business; it’s bad for the scraping community as a whole, as it can lead to stricter laws and less freedom for data collection activities.
By understanding and adhering to the legal and ethical considerations of web scraping, you can ensure that your scraping activities are above board and sustainable in the long term. The next sections will explore the practical aspects of web scraping costs and benefits, providing a full spectrum of insights into the practice.
Cost and Benefits
The Economics of Web Scraping
Web scraping, when done correctly, can be a significant economic boon to businesses and individuals alike. It’s a tool that can save hours of manual data collection, provide real-time insights, and fuel data-driven strategies. However, it’s not without its costs, both in terms of resources and potential risks.
Analyzing the Cost-Benefit of Web Scraping
When considering web scraping, it’s crucial to weigh the initial setup costs, including the development or purchase of scraping tools, against the potential benefits. Here’s how to approach it:
- Development Costs: Building a custom scraper requires an investment in software development, which includes time, expertise, and the potential cost of purchasing data scraping tools or services.
- Operational Costs: Running scrapers involves server costs, maintenance, and monitoring to ensure data quality and scraper performance.
- Risk Costs: Potential legal risks and the costs associated with data privacy compliance can add to the overall expense.
Cost-Saving Aspects of Web Scraping
Despite these costs, web scraping can lead to significant savings by:
- Automating Data Collection: Manual data collection is time-consuming and prone to error. Automation reduces labor costs and increases efficiency.
- Enhancing Competitive Analysis: Scraped data can provide insights into market trends and competitor behavior, allowing for more informed and timely business decisions.
- Improving Data-Driven Decisions: Access to more extensive and accurate data sets can lead to better business intelligence and outcomes.
The Return on Investment (ROI) of Web Scraping
Balancing the Scales
- Regularly Reviewing Scraping Practices: Continuously evaluate the effectiveness of your scraping practices and adjust as needed to ensure they remain cost-effective.
- Staying Informed on Legal Changes: Keep abreast of legal developments to avoid costly legal battles.
- Investing in Quality Tools: While free tools can be appealing, investing in quality scraping tools or services can provide better long-term value by reducing the likelihood of errors and downtime.
Web scraping is an investment with the potential for a high return. By understanding and managing the costs, and by maximizing the benefits through strategic use, organizations can leverage web scraping as a powerful competitive edge in their industry. The final section will offer tips and tricks to ensure that your scraping efforts are not only successful but also align with best practices and legal standards.
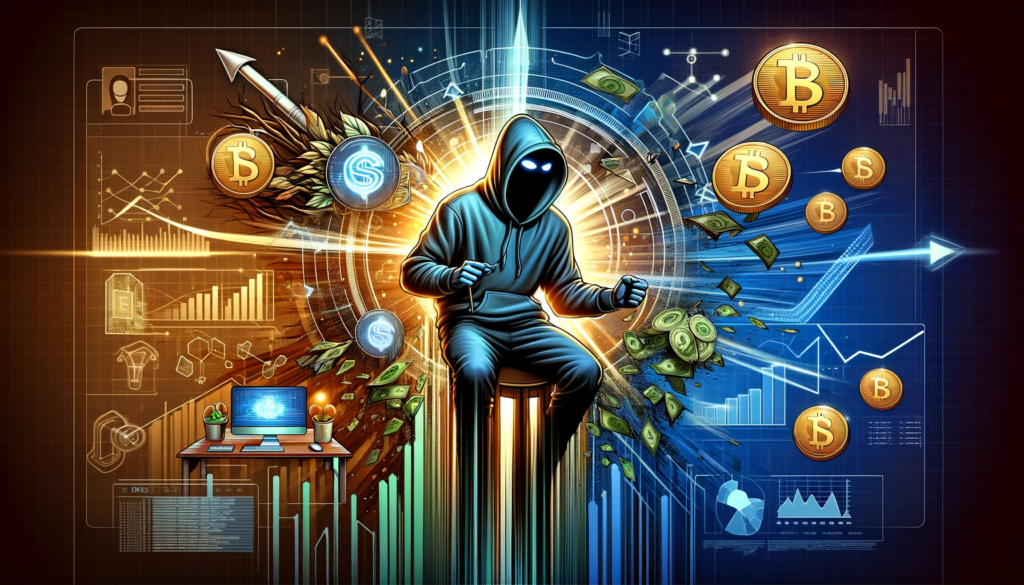
Monetization Strategies: Data as a Service (DaaS)
Web scraping is not just about data collection; it’s a gateway to innovative business models, especially through ‘Data as a Service’ (DaaS). This approach transforms raw web data into valuable insights, offering a range of monetization opportunities. Here’s how businesses are turning web scraping into profitable ventures:
Market Intelligence Reports:
- What It Involves: Gathering data on market trends, competitor analysis, consumer preferences, and industry shifts.
- Monetization Approach: Selling comprehensive reports or subscriptions to companies seeking up-to-date market intelligence.
Sentiment Analysis Services:
- What It Involves: Scraping social media and review sites for public opinion on products, services, or brands.
- Monetization Approach: Offering sentiment analysis as a service to businesses for brand monitoring and reputation management.
Lead Generation Services:
- What It Involves: Extracting contact information and business leads from various online sources.
- Monetization Approach: Selling leads to sales and marketing teams across industries.
E-commerce and Pricing Analytics:
- What It Involves: Tracking product prices, availability, and consumer reviews from various e-commerce platforms.
- Monetization Approach: Providing e-commerce businesses with insights to optimize their pricing and inventory strategies.
Real Estate Market Analysis:
- What It Involves: Scraping real estate listings, price trends, and property features.
- Monetization Approach: Offering data-driven insights to real estate investors and agencies.
Financial Market Insights:
- What It Involves: Collecting data on stock prices, market news, and economic indicators.
- Monetization Approach: Selling analysis and reports to investors and financial institutions.
Custom Research and Data Projects:
- What It Involves: Tailoring web scraping projects to specific client needs for unique data requirements.
- Monetization Approach: Offering bespoke data collection and analysis services.
SEO and Digital Marketing Insights:
- What It Involves: Scraping data on keywords, search engine rankings, and online marketing trends.
- Monetization Approach: Providing SEO agencies and digital marketers with actionable data to refine strategies.
Each of these avenues leverages the power of web scraping to turn vast online data into actionable, monetizable assets. By packaging scraped data into meaningful analytics, businesses can provide immense value to clients across various sectors, positioning themselves as essential partners in data-driven decision-making.
Web Scraping Tips for Beginners
Starting with web scraping can be overwhelming, but with the right approach, it can be made simpler and more effective. Here are some tips for those new to the field:
- Start Small: Begin with small, manageable projects to understand the basics before moving on to more complex tasks.
- Learn from the Community: Engage with online forums and communities. The shared knowledge from experienced scrapers can be invaluable.
- Use the Right Tools: Start with user-friendly tools like Octoparse or Instant Data Scraper before graduating to more complex scripts and frameworks.
Ensuring Quality and Efficiency
Quality and efficiency are key to successful web scraping. Keep these points in mind to maintain both:
- Validate Data as You Go: Regularly check the data you scrape for accuracy and completeness. This can save you from large-scale errors down the line.
- Stay Organized: Keep your code and data well-organized. This practice will make maintenance and updates much easier as your scraping projects grow.
- Optimize Your Code: Efficient code runs faster and reduces the load on both your system and the source website. Optimize your scripts to be as lean as possible.
Harnessing the Power of Web Scraping
Web scraping is an invaluable skill set in the digital era, offering the ability to turn the vast ocean of web data into actionable insights. This comprehensive guide has walked you through the essentials—from understanding the basics and the tools at your disposal to mastering the techniques and navigating the legal landscape.
As we’ve seen, web scraping is not just about technology; it’s about the strategic extraction and use of information. It’s a practice that, when done responsibly, can provide competitive intelligence, inform business strategies, and drive innovation. The key is to balance the technical aspects with ethical considerations and legal compliance, ensuring a sustainable approach to data collection.
Remember, the journey to effective web scraping is continuous. Websites evolve, new tools emerge, and legal frameworks change. Staying informed, practicing diligently, and adapting to new challenges are all part of the scraper’s path.
Whether you’re a business owner looking to monitor market trends, a developer creating the next generation of data-driven applications, or a researcher analyzing vast datasets, web scraping is a potent tool in your arsenal. Use it wisely, and you’ll unlock possibilities that were once beyond reach. With the tips and best practices outlined in this guide, you’re now ready to embark on your own web scraping ventures with confidence and skill.
Article Contents
- Introduction to Web Scraping
- Understanding Web Scraping
- Code Example: Basic Python Scraper
- The Mechanics of Web Scraping
- Web Scraping Tools
- Web Scraping with Python
- Effective Web Scraping Techniques
- Scraping Biggest Challenges
- Web Scraping Use Cases and Actual Real World Applications
- Legal and Ethical Considerations
- ROI of Web Scraping
- Conclusion